R's Mutate(): Data Transformation for Beginners
Data manipulation stands as a cornerstone of effective data analysis, and within the R programming language, the dplyr
package offers powerful tools for this purpose; dplyr
is a grammar of data manipulation, providing a consistent set of verbs that help you solve the most common data manipulation challenges. Central to dplyr
's capabilities is the mutate function in r
, which enables users to add new variables or modify existing ones within a data frame. Hadley Wickham, a prominent figure in the R community and the chief scientist at RStudio, developed dplyr
to streamline data workflows. Applying mutate()
in R is particularly useful when working with datasets from organizations like the World Bank, where creating new indicators from raw data is a frequent task.
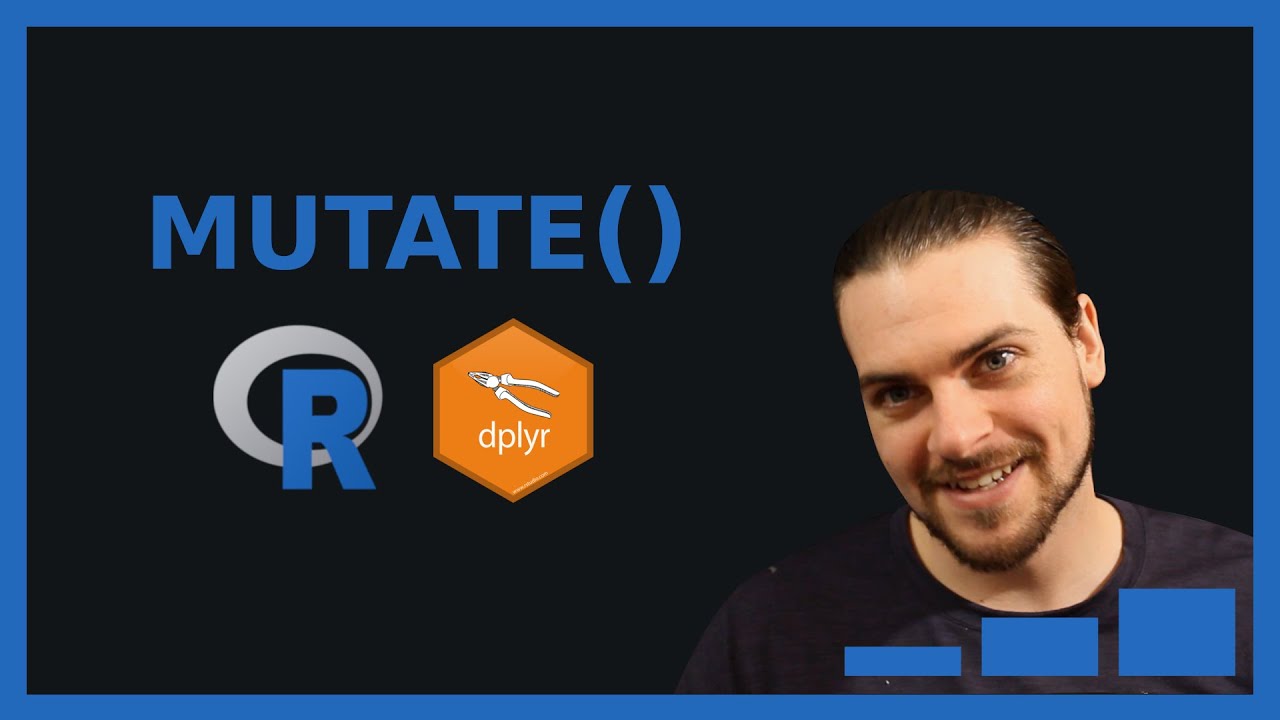
Image taken from the YouTube channel Dynamic Data Script , from the video titled dplyr::mutate() | How to use dplyr mutate function | R Programming .
The world of data analysis often feels like navigating a dense jungle of raw information. Before you can extract meaningful insights, the data needs to be tamed, reshaped, and refined. This is where mutate
, a powerful function from the dplyr
package in R, comes to the rescue.
mutate
is your go-to tool for adding new columns to your data frame or modifying existing ones with ease and efficiency.
It's a fundamental building block in any data transformation workflow, allowing you to create calculated variables, standardize values, and much more. Let's explore what makes it so essential.
mutate: Defining Data Transformation
At its core, mutate
is a function designed to transform your data.
Think of it as a sculptor's chisel, carefully shaping your dataset into a form that reveals hidden patterns and relationships.
More specifically, mutate
adds new columns to a data frame. Or it modifies existing columns based on calculations or transformations you specify.
This includes everything from simple arithmetic operations to complex conditional logic. The power is in your hands to mold your data into the shape you need.
Data Transformation and Data Wrangling Workflows
Data transformation is not just a preliminary step; it's an integral part of the entire data analysis process.
From initial data cleaning to creating features for machine learning models, the ability to efficiently transform your data is crucial.
mutate
streamlines this process by providing a clear and concise syntax for expressing complex transformations.
It enables you to perform calculations, create new variables, and manipulate existing columns within a single, readable command.
This function seamlessly integrates into data wrangling workflows, making data preparation tasks more manageable.
mutate and the tidyverse: A Powerful Combination
mutate
is a star player in the tidyverse
, a collection of R packages designed with usability and consistency in mind.
The tidyverse
promotes a coherent and intuitive approach to data science, making it easier to learn, use, and share your code.
By adhering to a common set of principles, the tidyverse
packages, including dplyr
(where mutate
resides), work harmoniously together.
This creates a seamless workflow from data import to visualization. mutate
fits perfectly into this ecosystem. It leverages the tidyverse
philosophy to provide a readable and efficient way to transform your data.
A Simple Example
To illustrate the basic usage of mutate
, consider a simple example:
Imagine you have a data frame called salesdata
with columns for revenue
and unitssold
. To calculate the average price per unit, you can use mutate
as follows:
library(dplyr)
salesdata <- salesdata %>%
mutate(averageprice = revenue / unitssold)
In this example, mutate
creates a new column called averageprice
by dividing the revenue
column by the unitssold
column.
The %>%
operator (the pipe operator) allows you to chain multiple dplyr
functions together, making your code more readable and concise.
This is a testament to the power and simplicity that mutate
brings to data manipulation in R.
Core Concepts: Data Transformation, Vectorization, and Tidy Data
The world of data analysis often feels like navigating a dense jungle of raw information. Before you can extract meaningful insights, the data needs to be tamed, reshaped, and refined. This is where mutate
, a powerful function from the dplyr
package in R, comes to the rescue.
mutate
is your go-to tool for adding new columns to your data frame or modifying existing ones, but to truly harness its power, you need to understand the core concepts that underpin its functionality: data transformation, vectorization, and the principles of tidy data. Let's explore each of these in detail.
Data Transformation: Shaping Raw Data into Usable Insights
Data transformation is the process of converting data from one format or structure into another. This is often essential to make the data suitable for analysis or visualization.
mutate
is instrumental in data transformation because it allows you to create new variables based on existing ones, thereby enabling you to derive insights that wouldn't be immediately apparent from the raw data.
Common types of data transformations include:
- Units Conversion: Converting measurements from one unit to another (e.g., inches to centimeters).
- Ratios: Calculating ratios or percentages from two or more variables.
- Standardization: Scaling and centering data to have a mean of 0 and a standard deviation of 1 (also known as z-scoring).
For example, suppose you have a dataset containing the height of individuals in inches, but you want to analyze the data in centimeters. You can use mutate
to create a new column with the height in centimeters:
library(dplyr)
data <- data.frame(height_inches = c(60, 65, 70, 72))
data_transformed <- data %>%
mutate(heightcm = heightinches **2.54)
print(data
_transformed)
This example showcases how mutate
transforms raw data into a more usable format for analysis.
Vectorization: Unleashing Efficiency in Data Manipulation
Vectorization is the process of performing operations on entire vectors (columns) of data at once, rather than looping through each element individually.
mutate
leverages vectorization to perform data transformations efficiently. This means that when you use mutate
to create a new column, the calculation is applied to all rows simultaneously, without the need for explicit loops.
Vectorized operations are significantly faster than traditional looping methods in R. This is because R is optimized for vectorized calculations. The performance benefits of vectorization become increasingly apparent as the size of the dataset grows.
Consider the following example comparing vectorized and looping approaches:
# Vectorized approach
data <- data.frame(x = 1:10000)
system.time(data <- data %>% mutate(y = x** 2))
# Looping approach
data <- data.frame(x = 1:10000)
system.time({
y <- numeric(nrow(data))
for (i in 1:nrow(data)) {
y[i] <- data$x[i] * 2
}
data$y <- y
})
You'll notice the vectorized version using mutate
is much faster.
Tidy Data: Structuring Data for Seamless Analysis
Tidy data is a standard way of structuring datasets where each variable forms a column, each observation forms a row, and each value forms a cell. This structure makes it easier to manipulate, analyze, and visualize data.
mutate
supports tidy datasets by allowing you to create new variables that conform to the tidy data principles. When you add a new column using mutate
, you're ensuring that each variable has its own column. Each observation has its own row.
For instance, imagine a dataset where you have separate columns for first name and last name, and you want to combine them into a single "full name" column. mutate
can help achieve this:
data <- data.frame(first_name = c("John", "Jane"), last_name = c("Doe", "Smith"))
data_tidy <- data %>%
mutate(fullname = paste(firstname, last_name, sep = " "))
print(data_tidy)
In summary, by understanding data transformation, vectorization, and tidy data, you unlock the full potential of mutate
and become a more effective data wrangler.
dplyr: The Home of mutate
The world of data analysis often feels like navigating a dense jungle of raw information. Before you can extract meaningful insights, the data needs to be tamed, reshaped, and refined. This is where mutate
, a powerful function from the dplyr
package in R, comes to the rescue.
mutate
isn't a lone ranger; it's part of a well-coordinated team, all residing within the dplyr
universe. Let's explore how dplyr
provides the environment where mutate
thrives and how other functions complement its capabilities.
dplyr
: More Than Just a Package
dplyr
is more than just a collection of functions. It's a grammar for data manipulation. This means it provides a consistent set of verbs that allow you to express complex data operations in a clear and readable manner.
Think of it like this: you wouldn't write a novel using random words. You need grammar to structure your thoughts. Similarly, dplyr
provides the grammar for structuring your data manipulations.
This grammar is built around a few core functions, each designed for a specific task. mutate
, of course, is the star of our show, but it's supported by a strong cast of supporting actors.
The dplyr
Family: Core Functions
While mutate
is essential for adding and modifying columns, it often works hand-in-hand with other dplyr
functions to achieve more complex data transformations. Here's a quick introduction to some of its closest relatives:
-
filter()
: Selecting Rows Based on Conditions.filter()
allows you to subset your data by selecting rows that meet specific criteria. For instance, you might usefilter()
to isolate data points within a specific date range or belonging to a particular category. -
select()
: Choosing the Columns You Need.select()
lets you pick the columns you want to keep. It’s handy for tidying up your data by removing irrelevant variables and focusing on the key information. -
groupby()
: Divide and Conquer with Data Grouping.groupby()
enables you to group your data based on one or more variables. This is a crucial step before performing calculations or transformations that need to be applied separately to each group. -
summarize()
: Condensing Data into Meaningful Summaries.summarize()
lets you calculate summary statistics for your data, such as means, medians, and standard deviations. It often works in tandem withgroup_by()
to generate summaries for each group in your data.
Working Together: mutate
and Friends in Action
The real power of dplyr
comes from combining these functions to create a pipeline of data transformations. Let's look at a simple example:
Imagine you have a dataset of sales transactions. You want to calculate the average transaction value for each customer, but only for transactions over $100.
Here's how you could achieve this using dplyr
:
library(dplyr)
sales_summary <- salesdata %>% filter(transactionamount > 100) %>% # Filter for transactions over $100 groupby(customerid) %>% # Group by customer ID summarize( averagetransaction = mean(transactionamount, na.rm = TRUE) # Calculate average ) %>% mutate( averagetransactionformatted = paste0("$", round(average
_transaction, 2)) #Format )
In this example, filter()
first selects relevant transactions. Then, group_by()
organizes the data by customer. summarize()
calculates the average transaction value for each customer.
Finally, mutate
adds a new column called "averagetransactionformatted" and format our previous result.
This is just a small illustration. dplyr
allows constructing chains of operations that transform your data from raw input into insightful, actionable results. It provides the structure, the language, and the tools to tell compelling stories with your data.
Integration with the tidyverse Ecosystem
The world of data analysis often feels like navigating a dense jungle of raw information. Before you can extract meaningful insights, the data needs to be tamed, reshaped, and refined. This is where mutate
, a powerful function from the dplyr
package in R, comes to the rescue.
mutate
isn't a lone ranger; it's part of a well-coordinated team. It thrives within the tidyverse
, a collection of R packages designed with a shared philosophy for data science. Let's explore how mutate
plays well with others, specifically readr
for data import and ggplot2
for visualization, showcasing its versatility.
dplyr
and the tidyverse
Philosophy
The tidyverse
isn't just a set of packages; it's a way of thinking about data. It promotes consistency, readability, and ease of use. dplyr
, as a core member, embodies these principles. Recognizing that dplyr
lives within this wider ecosystem provides a stronger foundation for understanding its potential.
The tidyverse
packages are designed to work together seamlessly. This integration streamlines the data science workflow, allowing you to move from data import to transformation to visualization with minimal friction.
mutate
and readr
: A Data Import Power Couple
Before you can transform data with mutate
, you need to get it into R. This is where readr
comes in. readr
provides functions for reading various data formats (CSV, TSV, etc.) into data frames.
readr
is designed to be fast and robust, automatically inferring column types. However, data rarely comes perfectly clean. Often, you'll need to perform initial transformations immediately after import, right after you’ve read the data.
Here, mutate
shines. You can chain readr
functions with dplyr
functions using the pipe operator (%>%
) to perform data cleaning and transformation in one go.
For example, imagine you're importing a dataset where a column representing currency is stored as a character string with a currency symbol. mutate
can be used to remove the symbol and convert the column to a numeric type during data import:
library(readr)
library(dplyr)
data <- readcsv("yourdata.csv") %>%
mutate(
price = parsenumber(price) # Uses readr's parsenumber to clean
)
This simple example demonstrates the power of combining readr
and mutate
to streamline data cleaning during the import process.
mutate
and ggplot2
: Preparing Data for Visual Storytelling
Data visualization is a crucial part of data analysis. It allows you to explore patterns, communicate insights, and tell a story with your data. ggplot2
, another tidyverse
package, provides a powerful and flexible system for creating visualizations in R.
However, ggplot2
requires data in a specific format. Often, you'll need to transform your data before you can create meaningful plots. This is where mutate
comes into play again.
mutate
can be used to create new variables or modify existing ones in preparation for plotting. For example, you might want to create a new variable representing a category based on a continuous variable.
Consider a scenario where you have data on customer ages and want to visualize customer distribution across age groups. Using mutate
, you can create a new variable that categorizes customers into age brackets:
library(ggplot2)
library(dplyr)
customer_data <- data.frame(age = sample(18:65, 100, replace = TRUE))
customer_data <- customerdata %>%
mutate(
agegroup = case_when(
age >= 18 & age <= 25 ~ "18-25",
age > 25 & age <= 35 ~ "26-35",
age > 35 & age <= 45 ~ "36-45",
TRUE ~ "46+"
)
)
ggplot(customer_data, aes(x = agegroup)) +
geombar()
In this example, mutate
prepares the customerdata
for ggplot2
by creating the agegroup
variable which is used to create the bars in the plot.
Real-World Applications and Examples
The combination of mutate
with other tidyverse
packages extends far beyond the simple examples above. Here are a few more scenarios:
- Feature Engineering: In machine learning,
mutate
can create new features by combining existing ones. - Data Aggregation: Use
group_by
andsummarize
(also fromdplyr
) withmutate
to create aggregate measures at different levels of granularity. - Time Series Analysis: Create lagged variables or calculate moving averages using
mutate
for time series forecasting.
By understanding how mutate
integrates with the broader tidyverse
ecosystem, you unlock a powerful toolkit for data manipulation, analysis, and visualization in R.
Key Contributors: Shaping the Landscape of Data Manipulation
Integration with the tidyverse Ecosystem
The world of data analysis often feels like navigating a dense jungle of raw information. Before you can extract meaningful insights, the data needs to be tamed, reshaped, and refined. This is where mutate
, a powerful function from the dplyr
package in R, comes to the rescue.
mutate
isn't a lone ranger; it's part of a larger ecosystem. The development and popularization of dplyr
and the broader tidyverse
is thanks to a dedicated group. These people helped make data manipulation more accessible and intuitive for countless analysts and researchers. Let's acknowledge some of the key figures and organizations whose contributions have been instrumental in shaping the landscape of data manipulation.
Hadley Wickham: Architect of the Tidyverse
Hadley Wickham is the name most synonymous with dplyr
and the tidyverse
.
As the primary author of these groundbreaking tools, his influence on the field of data science is undeniable. Wickham has not just provided the code but has also championed a philosophy of data analysis centered around clarity, consistency, and ease of use.
His work focuses on empowering users, regardless of their background. The goal is to perform complex data manipulations with relative simplicity. He built tools for everyone.
Shaping Accessible Data Manipulation
Wickham's key contribution lies in making data manipulation accessible to a broader audience. Before dplyr
, data manipulation in R often involved complex and sometimes convoluted code.
The tidyverse
introduced a more intuitive "grammar" for data manipulation. This allows users to express their intentions in a way that is closer to natural language.
This focus on readability and user-friendliness has lowered the barrier to entry for aspiring data scientists. It also enabled experienced analysts to be more efficient.
Garrett Grolemund: The Voice of Practical Data Science
While Hadley Wickham provided the architectural brilliance, Garrett Grolemund has played a vital role in teaching and popularizing data science skills. He makes data more approachable for learners.
Grolemund is best known as the co-author of "R for Data Science." It's a widely acclaimed book that serves as a comprehensive introduction to the tidyverse
.
His contributions extend beyond authorship, as he has actively promoted data literacy through various online courses and workshops. He has helped guide countless individuals on their journey into the world of data.
RStudio (Posit): Nurturing the Tidyverse Ecosystem
RStudio, now known as Posit, is the company that has provided crucial support and infrastructure for the tidyverse
ecosystem.
As the creator of the RStudio IDE, Posit has made R more accessible and user-friendly. The IDE is designed to improve the workflow for data analysis and visualization.
Posit is more than just a software company. It's a company that has actively invested in the development and maintenance of the tidyverse
packages. Posit provides resources, and fosters a vibrant community around these tools. This has contributed significantly to the widespread adoption and success of dplyr
and its companion packages.
Video: R's Mutate(): Data Transformation for Beginners
<h2>Frequently Asked Questions about R's Mutate()</h2>
<h3>What is the main purpose of the mutate function in R?</h3>
The main purpose of the `mutate` function in R, specifically within the `dplyr` package, is to add new variables or modify existing ones in a data frame. It allows you to perform data transformations based on existing columns and store the results as new columns in the same data frame.
<h3>How does mutate differ from simply assigning a new column using `$`?</h3>
While you can add a new column using the `$` operator (e.g., `my_data$new_column <- ...`), the `mutate` function in R offers several advantages. It's more readable, works seamlessly within the tidyverse workflow (especially with piping), and allows for multiple transformations in a single function call.
<h3>Can I use mutate to modify existing columns, or just create new ones?</h3>
The `mutate` function in R can both create new columns and modify existing ones. If you provide a new column name, it will be created. If you use the name of an existing column, its values will be overwritten with the result of your transformation.
<h3>Does the order of operations matter when using mutate to create multiple columns at once?</h3>
Yes, the order *does* matter when creating multiple columns in a single `mutate` call, because `mutate` evaluates expressions sequentially. Newly created columns are available for use in subsequent expressions within the same `mutate` function in R. So, if column B depends on column A (which is also being created in the same mutate call), make sure A is defined before B.
So, there you have it! You've now got a handle on the basics of mutate
function in R and how it can transform your data. Don't be afraid to experiment, try out different variations, and see what amazing things you can create. Happy coding!