Jackson Structured Programming: A Quick Start Guide
Jackson Structured Programming (JSP), a software development methodology, focuses on the data structures of a problem to derive the program structure. Michael Jackson, a British computer scientist, developed JSP in the 1970s to provide a systematic approach to program design. The methodology emphasizes creating structure diagrams that visually represent the hierarchical relationships within the input and output data. These diagrams are then transformed into a program structure that ensures the program correctly processes the data, making it easier to manage complex systems. The technique is beneficial when processing sequential data, as it provides a clearer path for development compared to the more traditional structured programming methods employed by organizations like IBM.
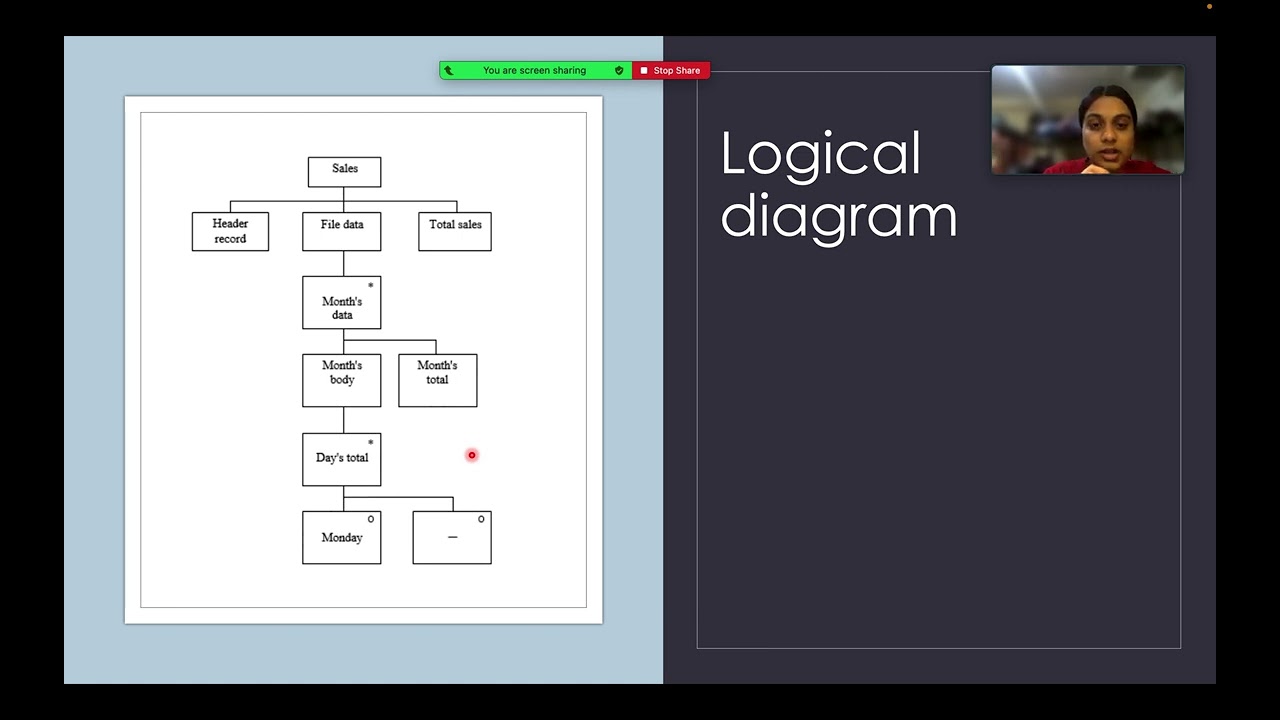
Image taken from the YouTube channel Prisha Mohan 22MIS0144 , from the video titled JACKSON STRUCTURED PROGRAMMING .
Jackson Structured Programming (JSP) stands as a robust program design methodology rooted in the principle that program structure should mirror the structure of the data it processes. This approach, developed by Michael A. Jackson, offers a systematic way to transform data structures into efficient and maintainable program code.
Defining Jackson Structured Programming
At its core, JSP is a program design method centered around the data. It emphasizes that the architecture of a program should be directly derived from the structure of the input and output data.
This method provides a visual and structured way to represent and manage program logic, ultimately leading to more predictable and understandable code. By mapping the program's structure directly to the data's structure, JSP simplifies the development process.
The Guiding Principle: Data Structure as Blueprint
The fundamental principle of JSP is that the structure of a program should faithfully reflect the structure of the data it manipulates. This principle simplifies the translation process from problem definition to code implementation.
By designing code to match the data structures, we can create more intuitive and error-resistant applications. This approach greatly enhances program clarity and reduces the cognitive load on developers.
Michael A. Jackson: The Architect of JSP
Michael A. Jackson, a prominent figure in software engineering, is the creator of JSP and the related Jackson System Development (JSD) methodology.
His work has significantly influenced structured programming practices, advocating for methods that align software development with the real-world structures it models. Jackson's focus on the importance of data structure in program design has left a lasting mark on the field.
His insights continue to inform contemporary approaches to software architecture and problem-solving.
Advantages of Using JSP
Choosing JSP offers numerous benefits, especially in the context of certain problem domains. The structured approach inherent in JSP significantly improves both maintainability and readability.
Programs designed with JSP tend to be easier to understand and modify. This clarity stems from the direct correlation between program structure and data structure.
JSP is particularly well-suited for data processing applications, where the input and output data have well-defined structures.
These applications often involve complex data transformations and reporting requirements, making JSP an ideal choice for managing complexity and ensuring accuracy. By leveraging JSP, developers can create robust and reliable systems that effectively handle data-intensive tasks.
Fundamental Concepts: Structure Diagrams and Control Flow
Jackson Structured Programming (JSP) stands as a robust program design methodology rooted in the principle that program structure should mirror the structure of the data it processes. This approach, developed by Michael A. Jackson, offers a systematic way to transform data structures into efficient and maintainable program code.
Defining Jackson Structured Programming (JSP) offers a unique and structured way to approach software development. Let's delve into the core concepts that make JSP a powerful tool for designing robust and understandable programs.
Structure Diagram: The Visual Language of JSP
The Structure Diagram is the cornerstone of JSP, serving as a visual representation of both the data and the program's architecture. Think of it as a blueprint that guides the entire development process.
It provides a clear and concise overview of how the program will process data, making it easier to understand and maintain. This visual approach sets JSP apart from other programming methodologies.
Components and Notation
Structure diagrams utilize a hierarchical tree-like structure. Each node represents a part of the data or a program construct.
- Rectangles typically represent sequence components.
- Circles denote iteration (repetition).
- Triangles signify selection (conditional branching).
These symbols, along with connecting lines, create a visual language that clearly communicates the program's logic.
Hierarchical Representation
The hierarchical nature of the structure diagram allows for a top-down approach to program design. Complex problems are broken down into smaller, more manageable sub-problems.
This hierarchical structure makes it easy to understand the relationships between different parts of the program. It promotes modularity and reduces the risk of errors. By representing data structures and program flow graphically, the structure diagram minimizes misunderstandings between developers and stakeholders.
Control Structures: The Building Blocks
Just like any programming language, JSP relies on fundamental control structures to dictate the flow of execution. These structures govern how the program processes data and performs operations.
Understanding these building blocks is essential for mastering JSP.
Sequence
Sequence refers to the linear execution of operations. Operations are executed in the order they appear in the structure diagram. This is the simplest control structure and forms the basis for more complex logic.
Selection
Selection, often referred to as conditional branching, allows the program to choose between different paths of execution based on a specific condition.
For example, if a customer's order total exceeds a certain amount, they might qualify for a discount. This logic can be elegantly represented using selection in a JSP structure diagram.
Iteration
Iteration enables the program to repeat a set of operations multiple times. This is crucial for processing collections of data or performing repetitive tasks.
For instance, reading through all the lines in a file or processing each record in a database table would require iteration.
Data Structure Diagram and Program Structure Diagram
In JSP, it's vital to distinguish between the Data Structure Diagram (DSD) and the Program Structure Diagram (PSD).
The DSD represents the structure of the input and output data. It outlines the hierarchical relationships between different data elements.
The PSD, on the other hand, depicts the structure of the program itself. Ideally, the PSD should mirror the DSD as closely as possible.
However, in some cases, data structures might be incompatible, requiring adjustments to the PSD. This is where techniques like interleaving and inversion come into play.
The goal is to create a PSD that effectively processes the data while maintaining a clear and understandable structure.
Elementary Operation: Defining Basic Actions
An Elementary Operation represents a fundamental action that the program performs. These operations are the smallest units of work within the JSP design.
Examples of elementary operations include reading a data record, performing a calculation, or writing output to a file. These operations are associated with specific nodes in the program structure diagram.
Identifying and defining elementary operations is a key step in the JSP design process. It helps to break down complex tasks into manageable components and ensures that the program performs the necessary actions to achieve the desired outcome.
The JSP Design Process: A Step-by-Step Guide
Fundamental Concepts: Structure Diagrams and Control Flow Jackson Structured Programming (JSP) stands as a robust program design methodology rooted in the principle that program structure should mirror the structure of the data it processes. This approach, developed by Michael A. Jackson, offers a systematic way to transform data structures into effective, well-organized program designs. Let's explore the design process in detail.
A Sequential Path to Structured Programs
The JSP design process is a meticulously defined, step-by-step methodology. It guides developers from initial problem analysis to the final transformation into executable code. Each step builds upon the previous one, ensuring a logical and coherent program structure.
Understanding the Problem and Data Structures (Input, Output)
The foundation of any successful JSP project lies in a thorough understanding of the problem. This begins with clearly defining the input data, its format, and its characteristics.
Simultaneously, you must identify the desired output, its structure, and its requirements. Analyzing both input and output is critical for shaping the program's architecture.
Creating Data Structure Diagrams
Once you comprehend the input and output, the next step involves visualizing them. Data Structure Diagrams (DSDs) are graphical representations illustrating the hierarchical organization of your data.
These diagrams depict sequences, selections, and iterations within the data. They act as blueprints, providing a clear and concise view of data relationships.
Creating the Program Structure Diagram
With the DSDs in place, you can begin crafting the Program Structure Diagram (PSD). The PSD mirrors the data structure diagrams, but it focuses on the program's structure.
Ideally, the PSD should align closely with the DSDs to ensure structural correspondence. This structural alignment is paramount for the effectiveness of JSP.
Listing Elementary Operations
Elementary operations are the basic, indivisible actions that the program must perform. These operations are low-level tasks, such as calculations, data manipulations, or input/output operations.
Listing all necessary elementary operations provides a comprehensive inventory of the program's functional requirements.
Allocating Operations to the Program Structure
This step involves strategically assigning each elementary operation to a specific node within the PSD. The goal is to integrate the operations seamlessly into the program's structure.
Careful allocation ensures that operations are performed in the correct order and at the appropriate time.
Transforming the Structure Diagram into Program Code (Program Transformation)
The final stage is transforming the PSD into actual program code. This transformation involves translating the diagram's structure and allocated operations into a programming language.
The structure diagram acts as a direct guide, enabling you to construct a well-organized and maintainable program.
Ensuring Structure Compatibility
Throughout the JSP design process, maintaining structure compatibility is of utmost importance. The structure of the program must be consistent with the structure of the data. When incompatibilities arise, techniques like interleaving or inversion become necessary to resolve these structure clashes, ensuring a cohesive and functional design. This dedication to structure compatibility helps prevent errors and ensures the program functions as expected.
Resolving Structure Clashes: Techniques for Incompatible Data
The JSP Design Process: A Step-by-Step Guide built upon the foundational elements of structured programming. A common stumbling block encountered during this process, however, is the structure clash. These clashes arise when the input and output data structures required by a program are fundamentally incompatible. This section delves into understanding these clashes and presents techniques like interleaving and inversion for their effective resolution.
Understanding Structure Clashes: The Root of the Problem
A structure clash, at its core, signifies a mismatch between the inherent structures of the data a program needs to process.
This mismatch can manifest in several ways, creating obstacles in translating the data structure diagrams into a coherent program structure.
Consider a scenario where one input file is sorted alphabetically while another is sorted numerically, and the program needs to merge them based on both criteria. The different ordering principles represent a structural incompatibility.
These incompatibilities hinder the direct mapping of data structures to program structures, violating a fundamental principle of JSP.
Failing to address these clashes can lead to convoluted, inefficient, and difficult-to-maintain code.
Common Techniques for Resolving Clashes
Fortunately, JSP offers specific techniques designed to resolve these structure clashes, allowing the program design to proceed smoothly. Two of the most commonly employed techniques are interleaving and inversion.
Interleaving: Managing Multiple Input Streams
Interleaving is a technique used when a program needs to process multiple input streams, each with its own distinct structure, in a coordinated manner. This is particularly useful when the different streams need to be processed in parallel or in a specific order.
Essentially, interleaving involves creating a program structure that can handle the different input streams concurrently, switching between them as needed. This switching is usually managed through flags and conditional logic that monitor the state of each input stream.
The advantage of interleaving is that it avoids the need to completely restructure or pre-process the input data.
However, it can increase the complexity of the program structure, as the control logic needs to manage multiple independent processes.
Properly implemented, interleaving allows a program to efficiently handle various inputs even when their inherent structures conflict.
Inversion: Restructuring Program Control
Inversion is a more drastic technique, employed when interleaving is insufficient or leads to excessive complexity. It involves fundamentally restructuring the program's control flow, essentially inverting the relationship between the program and its data.
Instead of the program actively reading and processing the data, the data source effectively "drives" the program. This is achieved by making the program a subroutine or a co-routine that is called by the data source whenever new data is available.
This technique is particularly effective when dealing with asynchronous input or when the data availability is unpredictable.
Inversion requires a deep understanding of both the data structure and the program's functional requirements.
It can result in a more complex program structure but can also significantly simplify the processing logic in scenarios where data availability dictates program execution.
In essence, Inversion allows the program to react appropriately and efficiently to the availability of data.
By strategically applying interleaving and inversion, developers can effectively address structure clashes and build robust, maintainable applications using Jackson Structured Programming.
Advanced JSP Techniques: Tackling Complexity
Resolving Structure Clashes: Techniques for Incompatible Data and The JSP Design Process: A Step-by-Step Guide built upon the foundational elements of structured programming. A common stumbling block encountered during this process, however, is the structure clash. These clashes arise when the input and output data structures required by a program are inherently incompatible. While interleaving and inversion offer robust solutions for many scenarios, more complex problems demand equally sophisticated techniques. This is where advanced JSP techniques, particularly Backtracking, come into play, enabling us to handle the uncertainty and ambiguous choices inherent in certain program designs.
Backtracking: Navigating Ambiguous Choices
Backtracking, in the context of JSP, is a technique employed when a program needs to explore multiple potential solutions or pathways to arrive at a correct output. It’s particularly valuable when faced with choices where the optimal path isn't immediately clear and the program might need to "undo" previous decisions.
Think of it as navigating a maze. At each intersection, you choose a path. If that path leads to a dead end, you "backtrack" to the last intersection and try a different route. This trial-and-error approach, systematically applied, eventually leads to the maze's exit, or, in our case, the desired solution.
How Backtracking Works in JSP
The implementation of backtracking within JSP relies on careful structuring and annotation of the program structure diagram. When a program reaches a decision point, it tentatively selects a choice.
This selection is marked, and the program continues.
If, later, it becomes evident that the initial choice was incorrect (perhaps leading to an invalid state or failure to meet requirements), the program "backtracks."
This involves undoing the effects of the initial choice and exploring an alternative. This process continues until a valid solution is found or all possibilities have been exhausted.
Key Considerations for Effective Backtracking
Several factors are crucial for implementing backtracking effectively:
-
Define Clear Choice Points: Identify the specific locations in the program where decisions need to be made and alternative paths explored.
-
Implement State Restoration: Ensure that the program can accurately revert to a previous state when backtracking is required. This often involves storing necessary data before making a choice.
-
Establish Termination Conditions: Define clear criteria for determining when a solution has been found or when all possible paths have been explored without success.
-
Manage Complexity: Backtracking can quickly become computationally expensive if not carefully managed. Employing heuristics and pruning techniques can help reduce the search space.
When to Consider Backtracking
Backtracking is particularly useful in scenarios such as:
-
Parsing and Lexical Analysis: When dealing with ambiguous grammars, backtracking allows the parser to explore different interpretations of the input until a valid parse tree is found.
-
Constraint Satisfaction Problems: When solving problems with multiple constraints, backtracking can be used to explore different combinations of variable assignments until a solution that satisfies all constraints is found.
-
Search and Optimization Algorithms: When searching for optimal solutions in complex search spaces, backtracking can be used to systematically explore different candidate solutions.
Backtracking: A Powerful Tool, Used Judiciously
Backtracking, while powerful, should be applied judiciously. It introduces complexity and can impact performance.
However, when facing problems with inherent uncertainty and ambiguous choices, backtracking provides a structured and systematic approach to finding solutions that might otherwise be elusive. By understanding its principles and applying it thoughtfully, programmers can significantly expand the range of problems they can effectively solve using Jackson Structured Programming.
Applying JSP in Practice: Real-World Examples and Best Practices
Resolving Structure Clashes: Techniques for Incompatible Data and The JSP Design Process: A Step-by-Step Guide built upon the foundational elements of structured programming. A common stumbling block encountered during this process, however, is the structure clash. These clashes arise when the input and output data structures have fundamentally different organizations, creating a mismatch that complicates the program's design.
Moving beyond theoretical concepts, let's explore how Jackson Structured Programming (JSP) can be effectively applied to solve real-world problems. JSP remains a valuable methodology, especially when dealing with complex data-driven applications.
Examples and Case Studies: JSP in Action
To truly appreciate the power of JSP, examining practical applications is essential. While purely academic exercises are useful for grasping the core principles, seeing how JSP tackles real-world scenarios highlights its continued relevance.
Consider a classic example: processing a sequential file of customer orders. Each order might contain multiple line items, and the file itself might be organized by customer, then by order date.
A JSP solution would begin by creating Data Structure Diagrams (DSDs) for both the input file format and the desired output report. The Program Structure Diagram (PSD) would then be carefully derived to mirror these data structures.
The PSD will handle complexities like iterating through customer records, processing each order, and then iterating through the line items within each order. This is the essence of JSP: structuring the program around the data.
Another example is a payroll system, which needs to process employee data and generate paychecks. This system would involve input files containing employee information, deductions, and hours worked, and it would produce pay stubs as output. JSP would ensure that the program structure aligns with the structure of this data, ensuring accurate and reliable processing.
While complete code examples would be extensive, illustrating portions of the DSD and PSD, even in a simplified manner, provides tangible insight. Even a partial structure diagram illustrates the fundamental benefits of visualizing data flow.
Tips and Best Practices for Applying JSP
Effective use of JSP goes beyond merely understanding the diagrams. Mastering the nuances of the methodology is crucial.
Here are a few key tips:
-
Start with a Clear Understanding of the Data: The more thoroughly you understand the input and output data structures, the easier it will be to create accurate and effective structure diagrams.
-
Keep Diagrams Simple and Focused: Avoid the temptation to overcomplicate your diagrams. Aim for clarity and conciseness.
Break down large, complex problems into smaller, more manageable subproblems.
-
Pay Close Attention to Structure Clashes: Identify potential structure clashes early in the design process and address them proactively using techniques like interleaving or inversion.
-
Embrace Iteration: JSP is often an iterative process. Don't be afraid to revise your diagrams and code as you gain a better understanding of the problem.
-
Validate your Structures: Always validate that your Program Structure Diagram accurately reflects the data flow and processing requirements.
JSP Today: Relevance for Modern Programmers
While JSP was originally developed for structured programming languages like COBOL, its core principles remain highly relevant in today's software development landscape.
Even in object-oriented or functional programming paradigms, the emphasis on data structure and program flow is valuable. Understanding the relationships between data and process is fundamental to good software design, regardless of the specific programming language or paradigm.
Professional programmers can leverage JSP principles in several ways:
-
Data Modeling: The DSDs created in JSP are valuable tools for data modeling, helping to understand the structure and relationships within data.
-
System Design: The approach of visualizing the system structure through PSDs is useful for high-level system design, providing a clear roadmap for development.
-
Problem Decomposition: Breaking down complex problems into smaller, manageable units based on data structures is a useful strategy for any development project.
-
Code Maintainability: By explicitly mapping program structure to data structure, JSP enhances code readability and maintainability.
-
Legacy Systems: Understanding JSP is useful for maintaining or modernizing legacy systems that were originally developed using this methodology.
While modern development methodologies often utilize more agile and iterative approaches, the core principles of JSP – understanding data structures, modeling program flow, and emphasizing a structured approach to development – remain powerful tools in a programmer's arsenal. Even if the complete JSP methodology is not adopted, its fundamental principles can significantly improve the quality and maintainability of modern software.
Video: Jackson Structured Programming: A Quick Start Guide
FAQs: Jackson Structured Programming: A Quick Start Guide
What is the main benefit of using Jackson Structured Programming (JSP)?
Jackson Structured Programming's primary benefit is creating software that accurately reflects the problem's structure. This makes programs easier to understand, maintain, and modify because the program design directly corresponds to the data it processes.
How does Jackson Structured Programming approach the design process?
JSP focuses on modeling the structure of the input and output data first. The program structure is then derived directly from these data structures. This contrasts with approaches that might prioritize algorithmic design initially. Jackson structured programming centers on the problem's data.
What are the basic diagram types used in Jackson Structured Programming?
JSP uses structure diagrams composed of sequence, selection (alternation), and iteration components. Sequence means steps happen in order. Selection indicates a choice between multiple paths. Iteration means a part of the process repeats.
What happens if the input and output data structures don't align perfectly in Jackson Structured Programming?
When input and output structures clash, Jackson Structured Programming introduces structure clashes. To resolve these, you often insert an intermediate file or perform program inversion, creating separate processes that communicate to bridge the structural differences.
So, that's Jackson Structured Programming in a nutshell! Hopefully, this quick start guide has given you a solid foundation. Now get out there, start structuring those problems, and see how much easier your coding life can become with Jackson Structured Programming. Good luck, and happy programming!